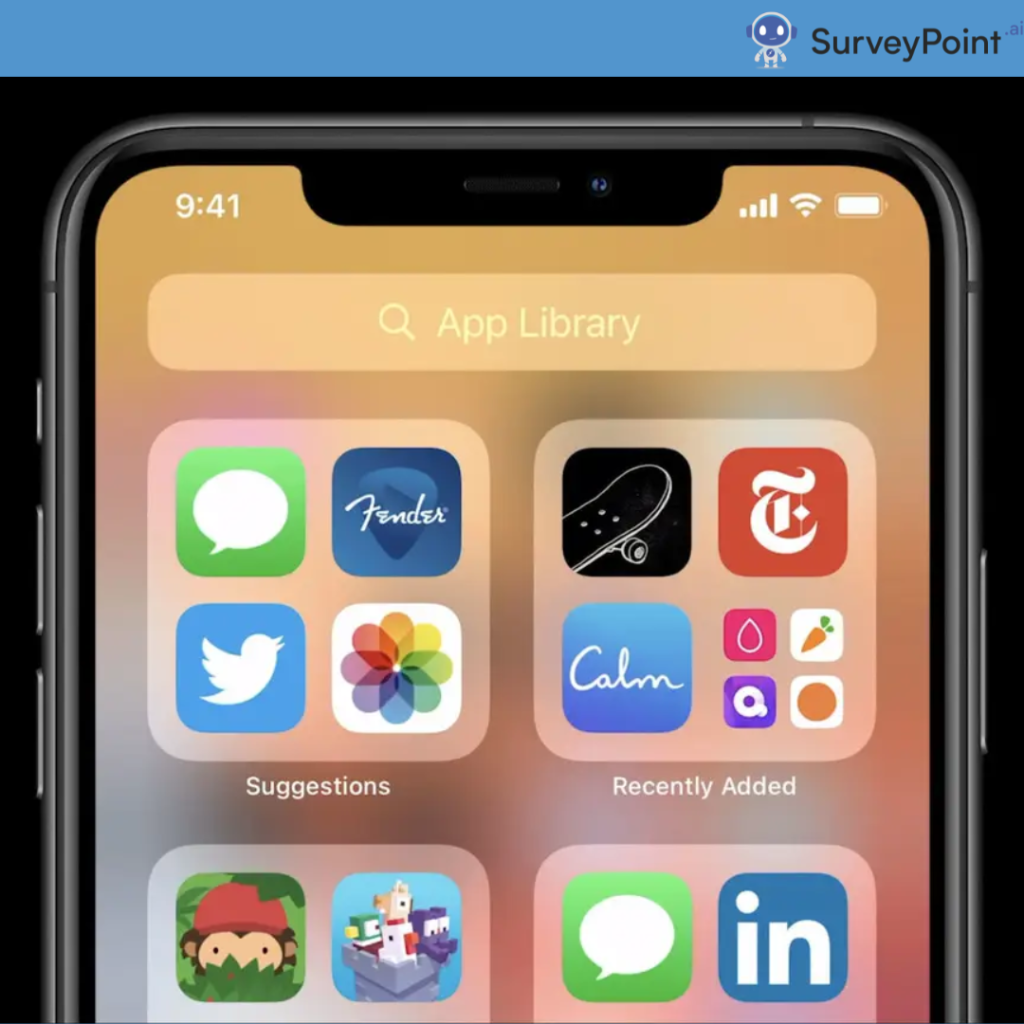
Creating an iOS app might seem intimidating, but with the right steps and tools, you can start building your app quickly—even if you’re a beginner. This guide will walk you through the basics, covering everything from setting up your environment to coding simple features.
Step 1: Set Up Xcode
The first step is to download Xcode, Apple’s integrated development environment (IDE) for building iOS applications. Xcode includes everything you need to create, test, and submit your app to the App Store. It’s available on the Mac App Store for free. Once you’ve downloaded it:
- Open Xcode and start a new project.
- Select “App” under the iOS category.
- Give your project a name, select Swift as your language, and pick “Storyboard” as the User Interface.
Step 2: Understand the Basics of Swift
Swift is the main programming language used for iOS development. It’s user-friendly, powerful, and relatively easy to learn for beginners. Here are a few fundamental concepts to get started:
- Variables: Store data. For example,
var name = "Hello World"
- Functions: Perform specific tasks. For instance,
func greetUser() { print("Hello, User!") }
- Classes: Define object templates with properties and functions.
Learning Swift basics can be done with Apple’s Swift Playgrounds app or through tutorials on websites like Ray Wenderlich and Hacking with Swift.
Step 3: Familiarize Yourself with Storyboard for UI Design
In iOS development, the Storyboard is where you design your app’s interface. It allows you to visually drag and drop elements like buttons, labels, and images onto your app’s screens.
- Open the
Main.storyboard
file in Xcode. - Drag elements from the Object Library (e.g., labels, buttons) onto the screen.
- Arrange and resize these elements as you see fit.
- To make buttons or images interactive, you’ll later connect these elements to your code using outlets and actions.
Step 4: Connect UI Elements to Code
To make your interface interactive, you need to connect UI elements in the Storyboard to your Swift code. Here’s how:
- Open the
ViewController.swift
file alongside your Storyboard by using the Assistant Editor. - Control-drag an element (like a button) from the Storyboard to the Swift file.
- Choose to create an “Action” if it’s something that should trigger a function, like a button tap, or an “Outlet” if it’s a variable that you’ll want to access and change later in the code.
For example, if you create an Action for a button, you might have something like this:
swiftCopy code@IBAction func buttonTapped(_ sender: UIButton) {
print("Button was tapped!")
}
Step 5: Write Basic Code to Add Functionality
Now, it’s time to code some functionality. Here are a few simple tasks you can implement:
- Change Label Text: When a button is tapped, change the text of a label.swiftCopy code
@IBOutlet weak var myLabel: UILabel! @IBAction func buttonTapped(_ sender: UIButton) { myLabel.text = "Hello, iOS Developer!" }
- Display an Alert: Show a pop-up message when the user interacts with an element.swiftCopy code
@IBAction func showAlert(_ sender: UIButton) { let alert = UIAlertController(title: "Welcome", message: "This is your first app!", preferredStyle: .alert) alert.addAction(UIAlertAction(title: "OK", style: .default, handler: nil)) present(alert, animated: true, completion: nil) }
These simple functions are great starters to get familiar with Swift syntax and connecting your UI elements.
Step 6: Test Your App in the Simulator
Xcode comes with an iOS Simulator that allows you to test your app on a virtual iPhone.
- Select your preferred iPhone model in the toolbar.
- Click the “Run” button (a play icon) in the upper-left corner of Xcode.
- Your app will build and launch in the simulator.
Testing helps you catch any errors and see if your code runs as expected before moving on to the next steps.
Step 7: Debugging Common Issues
Debugging is a crucial part of development. Here are common issues and how to solve them:
- Unresponsive Buttons: Ensure your button is correctly connected to an IBAction.
- UI Misalignment: Use the Auto Layout feature in the Storyboard to adjust elements to fit different screen sizes.
- App Crashes: Read the error logs in the Debug Console. Often, it’s due to a missing connection or incorrect syntax.
Debugging might feel overwhelming at first, but with practice, it becomes easier to spot and fix issues.
Step 8: Build More Features (Optional)
Now that you have a basic understanding, you can start adding more advanced features to your app:
- Navigation Controller: Set up navigation between multiple screens.
- Table Views: Display lists of data (like items in a checklist).
- Network Requests: Retrieve and display data from the internet using APIs.
Experiment with small projects or follow online tutorials to deepen your understanding.
Step 9: Testing on a Physical Device
While the iOS Simulator is great, testing on an actual iPhone or iPad provides a better feel for how your app performs. Here’s how to test on a physical device:
- Connect your iPhone to your Mac.
- Select your device in Xcode as the build target.
- Run the app as you did with the Simulator.
If it’s your first time running the app, you may need to set up a Developer Account through Xcode.
Step 10: Preparing for the App Store
Once your app is ready, it’s time to submit it to the App Store. Here are the main steps:
- Set Up Your App Store Connect Account: This is where you’ll manage your app’s listing.
- Archive Your App: In Xcode, go to Product > Archive to create a build that can be submitted.
- Upload to App Store Connect: Use Xcode to upload your archive to App Store Connect.
- Submit for Review: Fill in app details, such as the description and screenshots, and submit it for Apple’s review.
The review process can take a few days, so be prepared for possible feedback or revisions.
Conclusion
Building an iOS app is an exciting journey, even for beginners. By following these simple steps, you’ll be able to set up your development environment, code basic functionalities, and test your app. As you gain confidence, you can expand your skills by adding new features, exploring more advanced concepts, and, who knows, maybe even launching your app on the App Store!
Getting into iOS development might seem challenging, but taking it one step at a time can make the process enjoyable and rewarding. Happy coding! For more information checkout- surveypoint.ai